10 Simple Projects to Practice Software Development
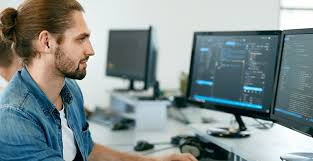
When it comes to learning software development, hands-on practice is one of the most effective ways to truly understand the concepts you're learning. It's easy to read tutorials and watch videos, but the best way to solidify your skills is by actually building something.
聽
In this guide, I鈥檒l walk you through 10 simple projects you can start working on today. These projects aren鈥檛 just about writing code; they鈥檒l help you practice key skills that every software developer needs鈥攚hether you're just getting started or have some experience already.
聽
Here are 10 projects that can help you improve your coding abilities while building something you can be proud of.
聽
1. Personal Portfolio Website
聽
A personal portfolio website is a project every developer should consider building early in their learning journey. Not only does it give you a place to showcase your skills, but it also teaches you essential web development principles.
聽
A personal portfolio is like a digital resume. You get to demonstrate your skills, show projects you've worked on, and highlight what you can do. Plus, it鈥檚 your opportunity to experiment with design and functionality.
聽
What You'll Learn:
- HTML & CSS: These are the basic building blocks of the web. HTML is used to structure your site, and CSS is used to style it.
- Responsive Design: Your portfolio needs to look good on all screen sizes, so you'll learn how to make it responsive.
- JavaScript: Use JavaScript to add some interactive elements. Maybe a contact form or an image slider.
Once completed, you鈥檒l have a website that serves as your professional online presence, helping future employers or clients see what you can do.
聽
2. To-Do List Application
聽
A To-Do List app is one of the simplest yet most powerful projects for practicing front-end logic and user interaction.
聽
This project is a perfect introduction to working with user input and managing tasks, which are key parts of any software application. In most apps, you鈥檒l be asked to manage data input and update the screen accordingly, just like you would with a to-do list.
聽
What You'll Learn:
- DOM manipulation: The DOM (Document Object Model) is what lets you interact with the HTML on the page. You'll learn to add, edit, and remove tasks dynamically.
- CRUD Operations: You鈥檒l work with Create, Read, Update, and Delete operations, which are essential for any data-driven app.
- JavaScript: This project will help you understand how to handle events like button clicks and how to store and retrieve data.
3. Calculator Application
聽
Building a calculator app may seem simple, but it teaches you to handle complex logic, user input, and basic arithmetic operations.
聽
Calculators are a great way to practice logic. You鈥檒l need to write code that handles input validation, performs operations, and updates the display in real time.
聽
What You'll Learn:
- Event-driven Programming: Every time a user clicks a button; your app needs to respond. This teaches you how to handle events in your code.
- Basic Math Operations: You鈥檒l practice building functionality for addition, subtraction, multiplication, and division.
- Conditional Statements: To ensure that calculations happen correctly, you鈥檒l need to use conditionals like if-else statements.
This project is also a great way to understand how your code interacts with user actions, which is key for building interactive applications.
聽
4. Weather App
聽
A Weather app is a fantastic project that helps you practice fetching real-world data and displaying it in a user-friendly way.
聽
By building a weather app, you鈥檒l get hands-on experience working with APIs, which allow your app to access data from external sources. Most modern apps rely on APIs for functionality, so this is a crucial skill.
聽
What You'll Learn:
- API Calls: You鈥檒l learn how to fetch data from an external service, like a weather API, and display it in your app.
- Handling Data: The data you receive will be in a specific format (like JSON), so you鈥檒l practice parsing and displaying it.
- Dynamic Content: Once you fetch the data, you鈥檒l need to update your app's UI in real time based on that data.
This project will give you confidence in integrating third-party services and handling data.
聽
5. Blog Platform
聽
Building a blog platform will teach you how to create both front-end and back-end components, making it an excellent project for full-stack development.
聽
A blog platform is a more advanced project that gets you thinking about data storage, user authentication, and dynamic content generation. It鈥檚 a real-world app that can help you understand how content management systems work.
聽
What You'll Learn:
- Database Integration: You鈥檒l need to store blog posts, comments, and user information in a database (e.g., MongoDB).
- User authentication: Allow users to register, log in, and post their own articles.
- Full-Stack Development: You鈥檒l work with both front-end and back-end technologies to create a complete app.
This project will help you become familiar with technologies like Node.js and Express, which are essential for full-stack development.
聽
6. Chat Application
聽
A chat application is a fun and challenging project where you build a real-time messaging platform.
聽
Building a chat app teaches you about real-time data and Web sockets, which are used for creating applications that need to update instantly (like chat apps, live updates, etc.).
聽
What You'll Learn:
- Real-Time Communication: With Web Sockets, you鈥檒l learn how to send and receive messages in real time.
- Backend Development: You鈥檒l learn how to manage user sessions and message storage.
- Event Handling: The app will need to respond to different events (sending a message, receiving a message, a user joining a room).
This is a great project if you want to dive deeper into building interactive, real-time applications.
聽
7. Expense Tracker
聽
An Expense Tracker helps users track their spending, and it鈥檚 a great project for learning about data persistence and user input.
聽
This app is a practical tool that teaches you how to manage data entry, perform calculations, and store information persistently (so users don鈥檛 lose their data).
聽
What You'll Learn:
- Local Storage or Databases: Learn how to store user input in local storage or a database for later retrieval.
- Data Validation: You鈥檒l need to check if the user enters valid data (e.g., numbers for expenses).
- Data Visualization: This project allows you to present the user鈥檚 data in a meaningful way (e.g., graphs or tables).
Building this app will give you a strong foundation in data management, which is essential for most software applications.
聽
8. Quiz Application
聽
A Quiz app is a fun way to practice creating forms, handling user input, and managing dynamic content.
聽
This project lets you practice creating quizzes with multiple-choice questions, keeping track of scores, and giving feedback to users based on their answers.
聽
What You'll Learn:
- Forms and Input Handling: Learn how to create forms and process the data that users input.
- Data Structures: You鈥檒l work with arrays and objects to manage the quiz questions and answers.
- Timers: Implementing a timer feature will help you practice working with time-based events.
This project reinforces skills that are useful for building educational apps or anything that involves quizzes.
聽
9. Recipe Book Application
聽
A Recipe Book app is a great project to learn about organizing data and creating dynamic, easy-to-navigate interfaces.
聽
This project will teach you how to build an app where users can input, search, and view recipes. It鈥檚 a great way to learn about managing data and building user-friendly interfaces.
聽
What You'll Learn:
- CRUD Operations: Users will create, read, update, and delete recipes, so you鈥檒l practice these fundamental operations.
- Search and Filter: Allow users to search and filter recipes based on categories or ingredients.
- Responsive Design: Ensure the app works well on different screen sizes.
This is a highly practical project that simulates the creation of apps you might use every day.
聽
10. Movie Database App
聽
A Movie Database app helps users find information about movies, and it's an excellent way to learn how to work with external APIs and display data.
聽
This app will give you experience in searching and filtering large sets of data, which is crucial for many real-world apps.
聽
What You'll Learn:
- API Integration: Fetch movie data from a third-party API and display it in your app.
- Search Functionality: Learn how to implement search and filter options to help users find movies.
- Pagination: Handle large amounts of data by paginating search results.
This project will enhance your skills in data fetching and UI/UX design.
聽
Conclusion
聽
These 10 simple projects are perfect for practicing your software development skills. Whether you're just getting started or you're looking to build more advanced projects, each one will help you improve your coding abilities and grow as a developer.
聽
Remember, the key to becoming a proficient developer is practice. So, pick a project, dive in, and start building. As you complete each one, you'll gain valuable experience and have a tangible product to show for your work.
聽
Don't worry about perfection鈥攆ocus on learning and improving as you go.