What Is Dependency Injection and Why Is It Helpful?
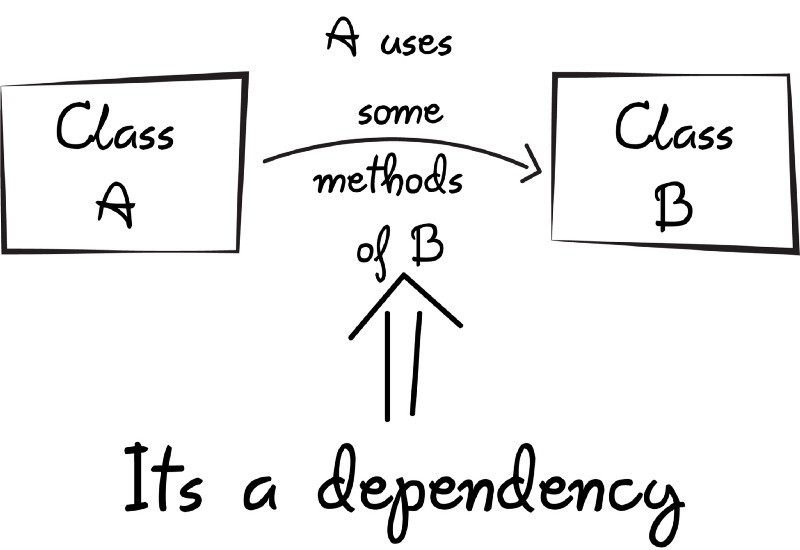
Dependency Injection (DI) is an approach that helps to manage how software components are connected. It is widely used because it allows for flexibility, easier maintenance, and reduces the complexity that comes with large codebases. In software development, it can be tricky to manage relationships between different parts of an application, especially when they rely heavily on each other. Dependency Injection was designed to make this process smoother, resulting in less tightly coupled code.
聽
The benefits of DI are clear when one considers how software applications evolve. As new features are added and requirements change, code can quickly become difficult to manage if dependencies between components are not handled well. DI introduces a structured way of managing these relationships, which helps to keep code flexible and easy to modify, without breaking the existing system.
聽
Why Is Dependency Injection Important?
聽
Dependency Injection is particularly useful when trying to keep code modular and scalable. It became a common solution because it allows components to be reused more easily. The key event that led to its popularity was the rise of object-oriented programming (OOP), which emphasized the separation of concerns. OOP encourages breaking code into objects with distinct responsibilities, but when those objects rely on each other too much, they become harder to manage. Dependency Injection allows these dependencies to be controlled externally rather than having objects manage their dependencies directly.
聽
Instead of an object being responsible for creating its dependencies, those dependencies are passed in from the outside. This shift is critical because it reduces the amount of hard-coded logic within a program. By introducing a system where dependencies are injected, objects become easier to test, update, and modify.
聽
An example:
# Without Dependency Injection
class Engine:
def start(self):
return "Engine started"
class Car:
def init(self):
self.engine = Engine() # Dependency created internally
def drive(self):
return self.engine.start()
car = Car()
print(car.drive()) # "Engine started"
In the example above, the Car
class directly depends on the Engine
class by creating an instance of it. If the Engine
class changes, the Car
class would need to be modified too. This makes maintenance harder as the system grows.
聽
How Does Dependency Injection Work?
聽
Dependency Injection can be handled in a few different ways, but the idea remains the same: instead of creating dependencies inside a class, they are provided externally. This can be done through constructor injection, setter injection, or interface injection. These methods allow flexibility in how dependencies are passed into the class.
聽
Constructor injection is the most common approach and occurs when the dependencies are passed as parameters to the class constructor. This method ensures that the object has all it needs when it is created, and there is no need to modify the object later.
聽
With Dependency Injection:
# With Dependency Injection
class Engine:
def start(self):
return "Engine started"
class Car:
def init(self, engine):
self.engine = engine # Dependency injected
def drive(self):
return self.engine.start()
engine = Engine()
car = Car(engine)
print(car.drive()) # "Engine started"
Here, the Engine
instance is provided to the Car
class from the outside. This makes the Car
class more flexible because any object that behaves like an engine can be used. If a new type of engine is needed, only the injected instance will change, without affecting the Car
class.
聽
The Impact of Dependency Injection
聽
The main effect of Dependency Injection is seen in how easier it makes testing and maintaining code. Software systems become more reliable and adaptable when DI is applied properly. During the development process, testing is a major part of ensuring that the system works as expected. Dependency Injection allows mock objects to be used for testing, which isolates parts of the system and ensures each part can be tested independently.
聽
For example, imagine testing the Car
class without actually starting a real engine:
class MockEngine:
def start(self):
return "Mock engine started"
mock_engine = MockEngine()
car = Car(mock_engine)
print(car.drive()) # "Mock engine started"
This code shows how easily a mock object can be injected, allowing for tests that don鈥檛 rely on a real engine. This approach became vital in large projects where testing real-world scenarios is impractical or expensive. Developers can run tests more quickly and with less effort by injecting mock dependencies.
Over time, Dependency Injection has been adopted in many programming frameworks. Popular frameworks like Spring for Java and .NET Core for C# rely heavily on DI to manage object creation and dependency resolution. These frameworks automatically inject the required dependencies, making the developer's job easier.
聽
The decision to use DI is often made early in the design process, as it influences how classes interact with each other. Although it requires some initial setup, the long-term benefits far outweigh the initial cost. Code becomes more modular, which is especially helpful in large teams where different developers may work on separate parts of the system.
聽
Why Dependency Injection Is Here to Stay
聽
Dependency Injection鈥檚 rise in popularity wasn鈥檛 accidental. As the software world grew and systems became more complex, it became necessary to find better ways to manage code. The immediate effect of DI is seen in cleaner, easier-to-read code that promotes loose coupling between classes. This helps developers avoid situations where one change in the system causes unexpected errors elsewhere.
聽
The long-term outcome of using DI is the creation of applications that can grow and evolve more smoothly. Systems become more scalable, and new features can be added without requiring a complete rewrite of the existing code. Teams also find it easier to collaborate, as the boundaries between different components are clearly defined.
聽
Dependency Injection continues to play an essential role in modern software development. Its ability to reduce code complexity, improve testability, and promote cleaner architecture ensures that it will remain a valuable tool for developers worldwide. Through its structured approach to managing dependencies, both new developers and seasoned professionals can benefit from the flexibility and clarity it provides.
聽
In summary, Dependency Injection is a practical approach that allows dependencies to be managed externally, resulting in code that is more flexible, modular, and easier to maintain. By injecting dependencies, software can evolve without breaking, making DI an essential technique in today鈥檚 development environment.